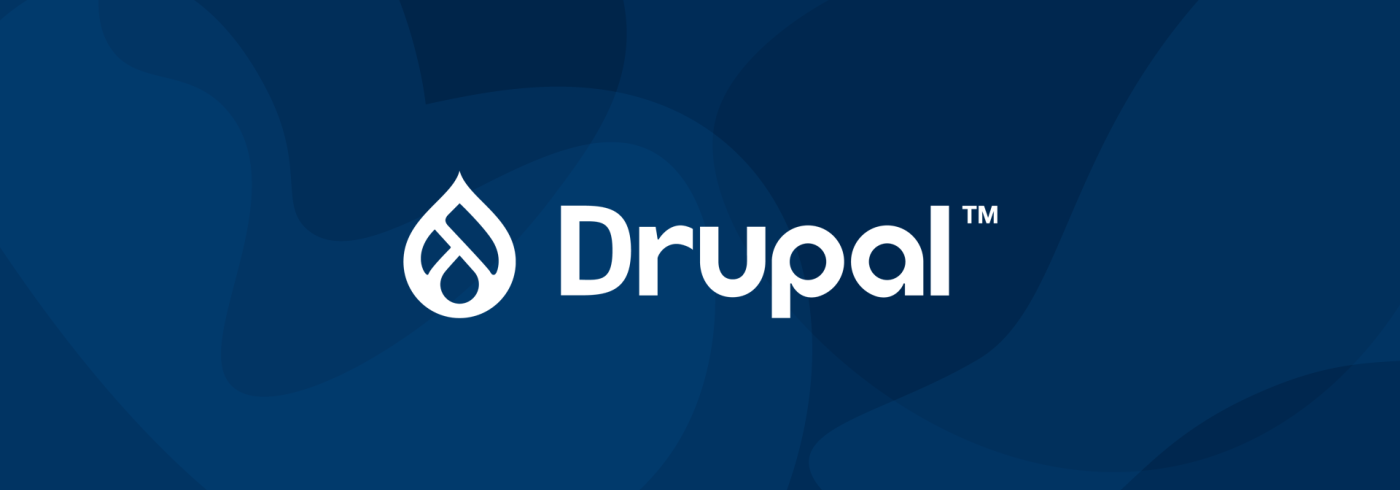
The Event Condition Action (or ECA) module is a set of Drupal modules that allows site builders to create custom workflows without having to write any code. Since the stable release of this module a few weeks ago I have been looking into it and see what makes it work.
The module works by listening for events, which it runs through one or more conditions, before running one or more actions. Using this module you can put together some amazing workflows without writing a single line of code.
The module has under 200 installs right now, and considering the quality of the module really isn't representative. It is well written, well thought out, and has a considerable number of unit tests that backup what the code is doing.
In this article, I will talk about installing the ECA module and go onto how to use it and some examples of it in use.
Installing ECA
To install ECA we first need to add it to our project, which can be done using composer.
composer require drupal/eca
The ECA module on its own just handles the actual events, conditions and actions. In order to configure the ECA module we need an interface. There are a couple of interfaces available, but we will use the BPMN.IO module.
BPMN.IO is a JavaScript framework that allows flow diagrams to be used and therefore gives the ECA module a well rounded interface. It is a separate requirement, but can also be installed through composer.
composer require drupal/bpmn_io
We can now install the ECA and BPMN.IO modules.
drush en eca bpmn_io
With those modules installed you can now visit the ECA admin page at /admin/config/workflow/eca and start building your workflows.
Out of the box the ECA module doesn't have a massive amount of integrations. There are quite a few sub-modules packaged with ECA that allow you to integrate with different parts of Drupal. Let's take a look at the modules available within ECA
ECA sub-modules
There are a few modules packaged with ECA and these can be enabled when you want to work with different parts of Drupal.
- ECA Access - Events and actions to control access on entities and fields.
- ECA Base - Base events, conditions and actions.
- ECA Cache - Actions to read, write or invalidate cache items.
- ECA Config - Configuration events.
- ECA Content - Content entity events, conditions and actions.
- ECA Form - Form API events, conditions and actions.
- ECA Log - Events and actions for Drupal log messages.
- ECA Migrate - Migrate events.
- ECA Misc - Miscellaneous events and conditions from Drupal core and the kernel.
- ECA Queue - Events, conditions and actions for queued operations.
- ECA User - User events, conditions and actions.
- ECA Views - Execute and export Views query results within ECA.
- ECA Workflow - Content entity workflow actions.
What module you enable depends on what it is that you want to do. For example, if you want to work with pages of content then you would enable ECA Content. This might seem like a lot of different modules, but this separation of concerns means that you don't need to install modules you would never use and prevents bloating the site.
Using ECA
The usage of ECA means also talking about using BPMN.IO since that's the simplest way to interface with creating and viewing workflows. Once you have decided what you want to do with ECA you can install the necessary sub-module and then start building your workflows.
Head over to the ECA admin page at /admin/config/workflow/eca and you should see an (empty) list of the available models.
Click on the "Add new model" button and you'll be presented straight away with a BPMN.IO interface.
I'll admit when I first saw this screen it was pretty intimidating. Although it does get a bit of time to get used to using it, there isn't really a lot going on. Most of the time you'll only need to deal with events (the circles), actions (the rounded rectangles) and conditions (the arrows that connect them). Gateways (the diamonds) can be used to chain multiple conditions together, or trigger multiple actions.
The panel in the right hand side is a dynamic inspector that will update depending on what you have selected. ECA also knows about how Drupal works so if you react to an entity event then ECA will transmit the entity forwards through the workflow.
What's great about this interface is everything can be annotated and labelled. Meaning, if you put together a workflow you can detail every condition and step through that workflow so it's much easier to see what is going on when you next edit it.
It's difficult to explain how to use this interface in detail without writing pages of meticulous (and boring!) text. Instead, I thought it would be best to show a few simple examples of the ECA module in action.
Example ECA workflows
I thought a good exercise would be to write some workflows I normally write code for. This was the ultimate aim of the module authors as they kept finding they would write the same code over and over again and built the ECA module to make their life easier.
Validate a form
Using the ECA From sub-module we can react to form events, including the validation of the form being run. By listening for this event we can detect if certain field values have been set and react accordingly.
For example, let's say we were running a news site and we absolutely didn't want a certain word to appear in our news articles. We could add a check that would throw a form error if the word "monkey" existed in the title.
This workflow consists of three elements.
- The event to trigger the workflow if the form validation event is happening.
- The condition to check on the presence of the word "monkey" in the title.
- The action to throw a validation error if the check is true.
Our created workflow would look something like this.
With this in place, when I try to create an article that has "monkey" in the title I get a validation error and have to re-word the title.
Of course, this is a simple example, but it could be extended to look at the values in more than one field and throw validation errors if certain conditions aren't met.
Hide a form element
Another very common thing that I write code for is hiding form elements. I have created countless hook_form_alter() hooks that tweak or change form elements, or change their access rights to hide them from certain users.
This is simple enough to do by just changing the access check on the form field, but we can easily build this in ECA without writing any code.
What we need to do here is install the ECA Form sub-module to manipulate the form and the ECA User sub-module to inspect the current user role. Then, it's a case of listening to the "Build form" action, checking the current user's role, and hiding the tags field if they aren't an "Editor".
This workflow again consists of just three elements.
- The event to trigger the workflow as the article page form is being built. We can put constraints on the event handler to ensure we are looking at a specific form.
- A connection that will check to see if the current user is a non-editor user. We do this by seeing if the user is an editor and then negating the output, which flips the logic.
- Finally, an action to alter the form and remove a specific field from the form, which in this case is the "tags" field.
This is the workflow that does this.
Now, when a non-editor user visits the article creation page they will not see the tags field. If we wanted to remove more fields we would just chain more actions from the end of the process to hide other fields on the form.
Redirect a user at login
As a more complex example I decided to show a workflow that redirected the user as they log into the site, but also performed a couple of other actions. Here are the actions I want to perform.
- If the user is an "editor" role, then redirect them to the /dashboard page
- If the user is an "author" role, then redirect them to the /dashboard page, but also display a message welcoming them to the site.
- Finally, take a look at the email address of the user and if it contains "example.com" then log this to the watchdog logs.
In order to get this workflow in place I needed to install the ECA User and ECA Log sub-modules to perform actions on the user and the log. Also, the ECA Base sub-module was installed as this provided a simple comparison operator that was useful in detecting if the user had an email address that contained a string.
There are a few ways in which to set up ECA to perform these actions, but here is the version I created.
This has two event listeners that both listen to the user logging into the site but perform different actions. The two checks then prevent those events progressing any further than they need to.
As the ECA module understands Drupal tokens we can print out a personalised welcome message using token replacement. The text "Welcome [current-user:display-name]!", will be replaced by the username of the user logging into the site.
The final action that logs a message to the database also uses tokens. Here, we setup a "contains" comparison between the token "[current-user:mail]" and the string "example.com". This means if the user's email address contains that string the event passes on and triggers the log being written.
This is a fairly custom workflow but it works really well. When I log into the site as different users with different email addresses the events are triggered and the actions are applied.
In addition it has saved writing any code on the site as everything is handled by ECA. This means changing the text of the welcome message can be done quickly without having to alter code.
Conclusion
What I have seen of the ECA module so far has been very impressive. As I mentioned before, the module has only recently been released, but it feels very well created and I haven't seen any errors or problems from it yet.
In fact, the main issue with the module is the learning curve. Getting into what module needs to be installed and how to plug together elements into something useful in the BPMN.IO interface is a little bewildering. After a couple of hours I was able to plug some components together and get workflows that altered forms, sent emails, redirected users and even changed content in pages.
One thing that might need to be improved is the comparisons only accept single values. For example, it is only possible to detect a single word in the form comparison whereas detecting a selection of words might be more useful.
The ECA module team has created a documentation site at https://ecaguide.org/ that details some of how to put together workflows and how to build your own ECA plugins. Handily, the relevant pages in the guide are linked from within the interface of the ECA module, which makes finding documentation on things really easy.
Once you have your ECA workflows setup you can export them just like any other item of Drupal configuration. They integrate well into the Drupal configuration workflow and allow you to properly test your more complex workflows before launching them.
There are also a number of third party modules that integrate ECA with different things, including one for Webform. Whilst many of these third party modules are in active development, it shows how easy it is to generate plugins for ECA that extend the functionality.
It's early days for this module, but I really feel it is the missing piece in the no-code approach to building your Drupal sites.