Managing PHP dependency versions can be complex, especially with development branches or custom patches. Composer aliases simplify this by allowing custom version names, enabling integration of development versions or patches without waiting for official releases
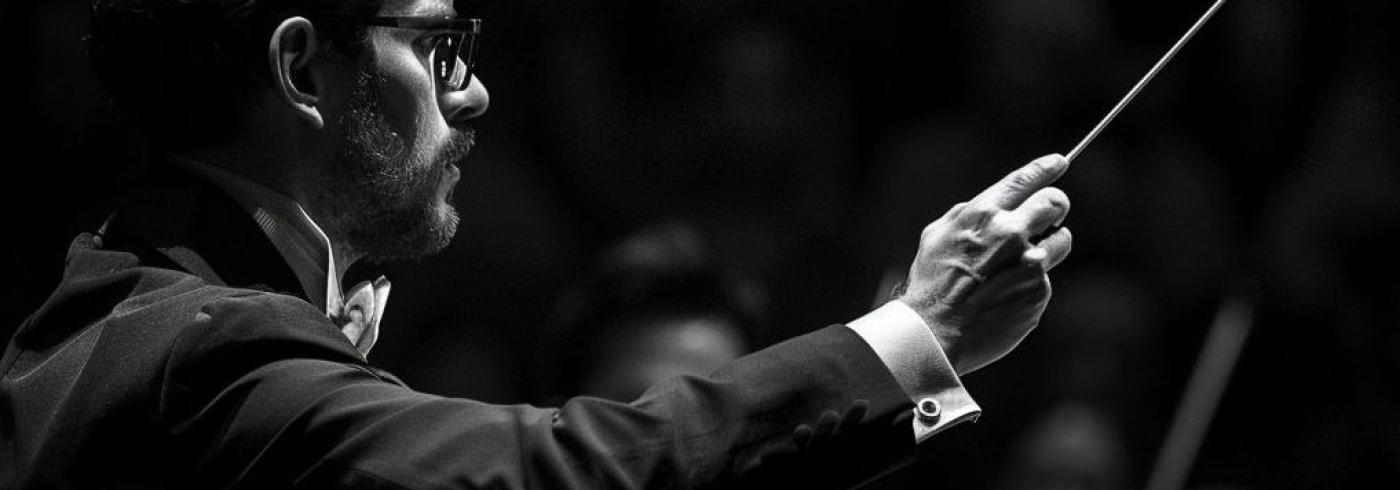
Composer aliases: managing versions with ease
Composer aliases allow you to specify custom version names for your dependencies. This feature is particularly useful when dealing with development versions or custom patches that you want to include in your project without waiting for an official release.
Practical use cases for composer aliases
- Development branches: Working on features that are in a development branch and want to use them as if they were part of a specific release.
- Hotfixes: Applying quick fixes without waiting for a new release from the package maintainer.
- Version consistency: Ensuring consistent version numbers across your project for better dependency management.
- Testing and development: Test new features or bug fixes from different branches or forks.
Setting up composer aliases
1. Define aliases in composer.json: To set up an alias, you need to define it in your `composer.json` file under the `require` section. This is especially handy when you want to use a specific branch or commit as if it were a stable release.
{
"require": {
"vendor/package": "dev-master as 1.0.0"
}
}
2. Using aliases for development: When working on a package that isn’t yet tagged with a stable version, you can alias the development branch to a version number that fits your project’s requirements.
{
"require": {
"vendor/package": "dev-feature-branch as 2.0.0"
}
}
3. Composer install and update: After defining the alias, run `composer install` or `composer update` to apply the changes.
composer install
Custom forks: controlling your dependencies
Custom forks provide a way to modify and control dependencies without waiting for changes to be accepted in the original package. This is particularly useful for critical bug fixes, custom feature additions, or experimental changes.
Combining aliases and forks
You can combine aliases with custom forks to maintain clean versioning and smooth integration into your projects. For example, if you create a feature in a custom fork, you can alias it to a version number that fits your project’s dependency constraints.
Practical example: testing a new feature in your module
Imagine you’re working on a PHP project that uses a library called codeenigma/mymodule
. The current stable version you are using is 1.0.0
. The developers of codeenigma/mymodule
are working on a new feature in a branch called feature/new-cool-feature
, and you want to test this feature in your project.
Here’s how you can use Composer aliases to achieve this:
Step 1: Original composer.json
Your original composer.json
file might look like this:
{
"require": {
"codeenigma/mymodule": "1.0.0"
}
}
Step 2: Modify composer.json to Use an Alias
You want to test the feature/new-cool-feature
branch without affecting the stability of your project. To do this, you will alias this branch to 1.1.0
.
Update your composer.json
to include the alias:
{
"require": {
"codeenigma/mymodule": "dev-feature/new-cool-feature as 1.1.0"
}
}
Step 3: Run Composer Update
Run composer update
to install the aliased version:
composer update
Step 4: Verify the Installation
Check the composer.lock
file to confirm that codeenigma/mymodule
has been updated to 1.1.0
:
{
"name": "codeenigma/mymodule",
"version": "1.1.0",
"source": {
"type": "git",
"url": "https://github.com/codeenigma/mymodule.git",
"reference": "refs/heads/feature/new-cool-feature"
}
}
This ensures that you are now using the feature/new-cool-feature
branch as version 1.1.0
in your project.
Step 5: Test the new feature
Now you can test the new feature in your project. If everything works as expected, you have successfully tested the new feature without disturbing the stable 1.0.0
version. If the feature is not stable or has issues, you can easily revert back to the original stable version by changing your composer.json
back:
{
"require": {
"codeenigma/mymodule": "1.0.0"
}
}
Benefits highlighted
- Flexibility: Switch between stable and development versions easily.
- Minimal disruption: Test new features without affecting the main codebase.
- Simple reversion: Revert to stable versions quickly if issues arise.
Conclusion
By using Composer aliases, you can efficiently manage and test different versions of dependencies in your PHP projects. This technique allows you to experiment with new features and fixes without compromising the stability of your main codebase. Give it a try in your next project to see how it can streamline your development process!